Every new year there’s always a bunch of articles about “Learn this in year 200X!”
But the problem is.. they’re always about libraries. Learn PrototypeJS. Learn jQuery. Learn Backbone. Learn AngularJS. Now it’s Learn React (or some React-derivative)
This stuff comes and goes!
Chances are, your job mostly dictates the libraries you’d use. Even if you do get to pick one, you’ll figure it out with your coworkers based on factors other than “some guy on the internet said we should use this”.
I’m not saying those articles are necessarily bad. They can be a useful look at what’s popular and what are some new interesting up and comers.
What I am saying is there are more useful things to learn beyond the cool library of the year.
Widely applicable skills for the win
While learning some specific library can be useful (especially if you want a job using that library), skills that can be applied more widely are much more useful in longer term.
When you were learning JavaScript, you didn’t just memorize the functions and what they did. You learned the language and how to use those functions to put together more complex things. You hopefully also learned how to find information on Google or MDN on them. When learning to build bigger applications, you didn’t just memorize the framework. You learned patterns like MVC, which continue to be useful beyond that original framework you used.
These are the kinds of things you want to learn – things which give you something you can keep using, even if your tools change. You can continue using many programming-related skills even if you change the language! You can’t say that about a library.
I’ve been programming for over 15 years. I started out with C, then basic HTML and CSS, and moved to PHP. Nowadays I’m building apps with JavaScript. Was what I learned with PHP wasted?
When I was working with PHP, I learned about libraries and frameworks for PHP. That knowledge is not very useful in JavaScript. But I also learned a number of other things as well, such as how the HTTP protocol works, web application security, test automation and so on. All of those are things that I still continue to benefit from even though I’m using a totally different language.
Heck, I can use that knowledge even when working with Haskell or some crazy thing that nobody understands at all.
—-
Below, I’m going to list a few suggestions on that kind of widely applicable skills you can pick up now, and continue getting benefits from for years to come.
Should you learn all of them? Maybe. But you don’t have to – pick one which you find most interesting, or which you think you’d get most benefits from.
Web application security
My favorite hacking gif
Let’s start with the one which is possibly the most important one, especially with today’s world of ever-increasing surveillance and bigger and worse data breaches in companies.
The topic of web application security does not receive enough attention. Thankfully many of today’s libraries used to build apps integrate some measures directly into them, but as a developer, you still need to be aware of what problems exist in order to truly mitigate them.
To list a few…
- SQL injection
- XSS or Cross-Site Scripting vulnerabilities
- CSRF or Cross Site Request Forgery exploits
- Many more!
I remember learning about these 5-10 years ago, and they are still a thing. I suspect they will continue to be a thing, along with fun brand new threats, for the foreseeable future.
Security concerns are of critical importance to know for all software developers.
Resources:
- The OWASP wiki is the single best source of information on web app security, including tips on prevention
- Technically not a resource, but shows how security issues can arise from things you wouldn’t even consider at first: The Line-of-Death
Functional Programming
Functional programming is the new hotness, and some advocate going really, really head on into functional programming in JavaScript.
But frankly, that’s overkill.
JavaScript is not a language like Haskell. If you try to shoehorn too many things from the “hardcore” FP world into it, things are going to be kind of terrible.
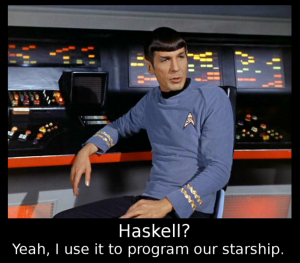
So why am I including functional programming here?
In general, functional programming allows us to write our programs in ways which make them more general and easier to reason about. Meaning, our code becomes more reusable and we need less code to perform the same tasks, and because the code is easier to reason about, it’s easier to understand what’s happening and as a result we’ll have fewer bugs.
While JavaScript isn’t suitable for “hardcore” FP, we can apply certain aspects of functional programming without problems. Those are some of the aspects that help us write code that’s more reliable and easier to understand and reason about. That sounds pretty good, right?
Here’s two examples:
- Pure functions: The idea that a function does not have side-effects or change any external state. Given certain parameters, they always return the same result, regardless of application state.
This means we can trust that the result is always going to be correct, and that they won’t change anything unexpectedly. They are also much easier to reuse elsewhere in the code as a result.
- Immutable data: Normally in JavaScript, data is mutable. Meaning you can change it at will. However, being able to change data at will can easily cause bugs.
I’m sure you’ve heard that global variables are bad. But why are they bad? Because anyone can change them from anywhere, which can easily lead to bugs. The idea with immutable data is similar, but on smaller scale.
By making data immutable, we can reduce the amount of bugs that occur because someone changed it, when we didn’t think it would change. This can especially be a problem with objects and arrays, since they are copied by reference in JavaScript.
There are more things about functional programming which can be helpful in day to day “non functional” programming too. If you learn to make use of the two concepts above, great! But if you really want to get a whole new perspective into how you can structure and think about your programs, I’d suggest learning Haskell or Elm. While they might not be immediately practical, Haskell especially requires a completely different approach which can be extremely valuable to understand even when writing code in JavaScript.
Resources:
- Kyle Simpson’s Functional-Light JavaScript book (free to read online) introduces many FP concepts without drowning you in all the crazy functional programming lingo
- An alternative FP JavaScript resource, the Mostly Adequate Guide
- Elm is a functional compiles-to-JS language which is easy to learn and practical
- PureScript is a Haskell-like language that compiles to JavaScript. May be harder to learn than Elm
- If you’re looking to really get into FP, go straight to learning Haskell. Note: You might not be immediately productive with it, it certainly took me a while to get there
Test automation
If you’ve read anything I’ve written before, you know I’m a big fan of test automation.
Manual testing is such a chore, but you can’t really leave your code untested. Thus, test automation to the rescue!
- You can trust your code works even if you change it, with less manual testing chores
- Fewer bugs, less debugging nightmares, more fun things like adding new features
- Test-driven development can even help improve your overall code quality
Been saying this for years but so many devs are in denial pic.twitter.com/KGqciUiOHS
— Chris Hartjes (@grmpyprogrammer) December 15, 2016
Test automation encompasses several different sub-topics:
- Unit testing
- Test-Driven Development
- Functional Testing / Browser Testing
- etc.
Similar to functional programming, the challenge here is the learning part, and then knowing how to apply what you’ve learned into practice.
Resources:
- If you want to quickly get started with automatic your JavaScript testing, get my JavaScript testing quickstart guide.
Communication skills / sales / marketing
Woah woah woah I thought this was about developer skills!
Well yes, it is. I know a lot of developers are mainly interested in just writing code and building cool stuff. I certainly was!
However as a hobby I helped a lot of people on IRC solve their programming problems. I also started writing a blog over 10 years ago. I was always interested in arguing my points to death. As a result, I learned how to talk about software issues with people with different backgrounds, ethically convincing people that my ideas work, and so on. As a result, I often became the “go to guy” at work whenever there were issues or other things. A good example of this was I was once hired initially as “just” a dev, but almost immediately got moved into a team tech lead type role.
There’s plenty of cases where these skills come in handy:
- You want to refactor some code, but you’re told there’s no time
- You want to add unit tests, but you’re told “let’s do it later” (read: never)
- You have this great idea for a feature or improvement, but nobody really cares
- Your coworker writes garbage code but you can’t get them to do anything about it
- etc.
Do you see where I’m going with this?
There’s a ton of cases as a developer where you have to sell your boss your idea. In a job interview, you have to market you. To get your coworker to stop writing garbage code, you need to communicate it to them in a way that they care about it too.
Communication skills are especially important if you want to advance in your career to positions with more responsibility or management.
Unfortunately for this topic I don’t have any resources to suggest. If you’re interested in this, subscribe to my newsletter with the form at the end of this article, and drop me a line – I’d be more than happy to discuss this further.
I can however make some suggestions:
- Consider helping others solve their problems. Go on IRC, Gitter, Stack Overflow, and answer questions. This forces you to learn to better understand potentially vague questions, ask good questions to get more info, and then answer them in a way which is understandable by people with varying levels of expertise.
- Consider writing a blog. Even if you write very randomly, it helps you learn to better structure your thoughts even on complicated topics. You’ll learn to simplify concepts so they are easier for others to learn and understand, which is again valuable when discussing things at work with people from different (possibly even non-technical!) backgrounds
Conclusion
While learning new libraries and frameworks is often needed for day to day work, you often don’t need to learn them up front.
Instead, it’s more valuable to focus on learning big picture topics which you can continue making use of for many many years to come – even if you decide to jump from using one language to another, like I went from PHP to JavaScript.